Web Widget (MeaWallet)
Introduction to the Web Widget
Part of our embedded scope is the access to sensitive card data. To access such card data, like PAN and CVV, it is mandatory to use a web widget. The reason being that this type of card data needs special protection according to PCI-DSS guidelines. If you are yourself PCI-DSS certified, you may also use the API to access sensitive card data.
There are several options to adjust the web widget to you UI/UX needs.
How to Show Sensitive Card Data via Web Widget
As mentioned before, a special web widget is needed. It renders the card data as shown in the following example.
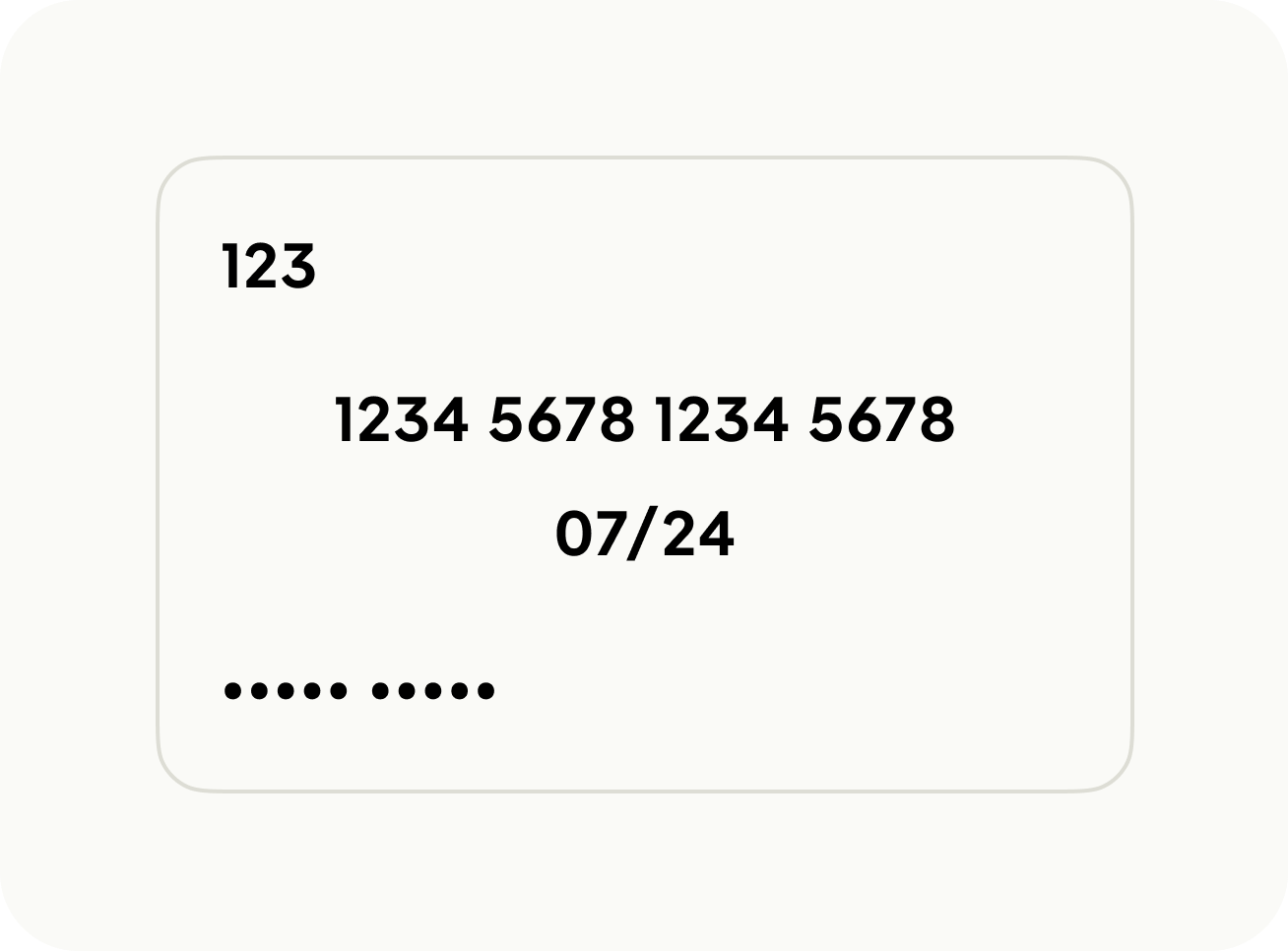
The web widget can be embedded into your frontend via an iframe
, which shows the card data for about 60 seconds. After that, the card details are hidden again.
<iframe
src="https://public.test.meawallet.com/easylaunch?var=<RANDOM>&data=<DATA>"
title="Pliant Credit Card"
width="400px"
height="225px"
frameBorder="0"
/>
Completed with data, the iframe
might look like this:
<iframe
src="https://public.test.meawallet.com/easylaunch?var=658647&data=eyJhcGlLZXlJZCI6IkFQSV9LRVlfSUQiLCJhcGlLZXkiOiJBUElfS0VZIiwiY2FyZElkIjoiMTIzNDU2Nzg5Iiwic2VjcmV0IjoiMDAxIzEyMzQ1Njc4IiwiZGVzdGluYXRpb24iOiJJU1NVRVIiLCJzdHlsZVVybCI6IiIsImZpZWxkTmFtZXMiOiJ7XCJwYW5cIjogXCJQQU46XCIsIFwiZW1ib3NzXCI6IFwiQ2FyZGhvbGRlcjpcIiwgXCJleHBcIjogXCJFeHBpcnk6XCIsIFwiY3Z2XCI6IFwiQ1ZWOlwifSIsImxvYWRlciI6ImRvdHMifQ=="
title="Pliant Credit Card"
width="400px"
height="225px"
frameBorder="0"
/>
Random Data
The random number value should be passed to the var
variable to avoid browsers caching card data values or styles.
const random = Math.floor(Math.random() * 1000000);
Data Payload
The actual data payload which is needed to show the specific sensitive card details is transmitted via the data
variable. It is a base64
encoded JSON payload.
{
"apiKeyId": "API_KEY_ID",
"apiKey": "API_KEY",
"cardId": "123456789",
"uniqueToken": "001#12345678",
"styleUrl": "",
"fieldNames": "{\"pan\": \"PAN:\", \"emboss\": \"Cardholder:\", \"exp\": \"Expiry:\", \"cvv\": \"CVV:\"}",
"loader": "dots"
}
Which will then be attached to the URL as an base64
encoded string:
ewogICJhcGlLZXlJZCI6ICJBUElfS0VZX0lEIiwKICAiYXBpS2V5IjogIkFQSV9LRVkiLAogICJjYXJkSWQiOiAiMTIzNDU2Nzg5IiwKICAidW5pcXVlVG9rZW4iOiAiMDAxIzEyMzQ1Njc4IiwKICAiZGVzdGluYXRpb24iOiAiSVNTVUVSIiwKICAic3R5bGVVcmwiOiAiIiwKICAiZmllbGROYW1lcyI6ICJ7XCJwYW5cIjogXCJQQU46XCIsIFwiZW1ib3NzXCI6IFwiQ2FyZGhvbGRlcjpcIiwgXCJleHBcIjogXCJFeHBpcnk6XCIsIFwiY3Z2XCI6IFwiQ1ZWOlwifSIsCiAgImxvYWRlciI6ICJkb3RzIgp9
Data Parameters
Parameter | Description | Required |
---|---|---|
cardId | The token value from the card details endpoint for the specific card you want to show. | X |
uniqueToken | Represents a TOTP token which is valid for this one call. See details below for generation of such a key. The token consists of three parts:1. Key ID (3 digits) - Used to select the correct secret key. 2. Separator (1 symbol) - Separator of key ID and TOTP. 3. TOTP (8 digits) - Time-based One Time Password. | X |
apiKeyId | The public key fingerprint. Shared with you during onboarding to this API feature. | X |
apiKey | The public key. Shared with you during onboarding to this API feature. | X |
fieldNames | This string-escaped JSON allows to customize card value field names. Empty (no field names) by default. | |
loader | The style of the loader. Available values - bars , dots , spinner . dots by default. | |
styleUrl | A URL to custom style CSS. |
Generation of the uniqueToken
uniqueToken
The uniqueToken
that is needed to make the web widget show the correct card data is a TOTP, or time-based one-time password (see Wikipedia for details).
The generation of such token can be either implemented by the Partner themselves or the provided library is used, see the following guide for implementation details.
URLs
Environment | URL |
---|---|
Test | https://public.test.meawallet.com/easylaunch |
Production | https://mcd.meawallet.com/easylaunch |
Styling of the Web Widget
A custom appearance of the card view can be achieved by passing styleUrl
to custom CSS hosted on a partners server. Everything is allowed to be customized! You can even change the order of the shown attributes via CSS and for instance show the card in a vertical layout instead of a horizontal layout. Design-wise you are 100% to style the card appearance.
The HTML of the card web widget is structured as follows. Which can then be styled according to the different CSS classes used in the HTML.
<div id="app" class="mea-easy-launch-app">
<div class="mea-easy-launch-container">
<div class="mea-data mea-pan-result">
<div class="mea-field-name mea-field-pan-name">PAN:</div>
<span class="mea-field-value mea-field-pan-value">1234 5678 1234 5678</span>
</div>
<div class="mea-data mea-cardholder-result">
<div class="mea-field-name mea-field-cardholder-name">Cardholder:</div>
<span class="mea-field-value mea-field-cardholder-value">Jane Hopkins</span>
</div>
<div class="mea-data mea-expiry-result">
<div class="mea-field-name mea-field-expiry-name">Expiry:</div>
<span class="mea-field-value mea-field-expiry-value">07/27</span>
</div>
<div class="mea-data mea-cvv-result">
<div class="mea-field-name mea-field-cvv-name">CVV:</div>
<span class="mea-field-value mea-field-cvv-value">123</span>
</div>
</div>
</div>
The default style that applies:
.mea-easy-launch-app .vld-overlay .vld-icon svg {
fill: white;
}
.mea-easy-launch-app {
background: linear-gradient(30deg, rgba(1, 143, 219, 1) 0%, rgba(249, 95, 3, 1) 95%);
border-radius: 15px;
color: white;
font-family: Helvetica, sans-serif !important;
height: 100%;
position: absolute;
width: 100%;
}
.mea-easy-launch-container {
display: flex;
flex-direction: column;
font-size: 20px;
height: 100%;
}
.mea-easy-launch-container .mea-data:nth-child(1) { order: 2; }
.mea-easy-launch-container .mea-data:nth-child(2) { order: 4; }
.mea-easy-launch-container .mea-data:nth-child(3) { order: 3; }
.mea-easy-launch-container .mea-data:nth-child(4) { order: 1; }
.mea-cvv-result {
padding-left: 20px;
padding-top: 15px;
text-align: left;
}
.mea-cardholder-result {
margin-top: auto;
padding-bottom: 15px;
padding-left: 20px;
}
.mea-pan-result {
padding-bottom: 10px;
padding-top: 45px;
}
.mea-expiry-result,
.mea-pan-result {
text-align: center;
}
Allowing to copy data from the iFrame
You can use an advanced feature to allow easy copying of credit card data for the end user.
allow="clipboard-write <environment URL>"
So for example like this:
<iframe
src="https://mcd.meawallet.com/easylaunch?var=<RANDOM>&data=<DATA>"
title="Bank Card"
width="400px"
height="225px"
frameBorder="0"
allow="clipboard-write https://mcd.meawallet.com/easylaunch"
/>
Further Information
For more information on this topic contact [email protected]
Updated 7 months ago